C++のSTL(Standard Template Library、標準テンプレートライブラリ)でvectorのメンバーを削除する方法の紹介です。
目次
C++でループしてvectorのメンバーを削除
C++のSTL(Standard Template Library)でループ処理の途中でvectorのメンバーを削除することはよくあります。
サンプルコードは以下の通りです。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
#include "stdafx.h" #include <stdint.h> #include <vector> #include <string> #include <iostream> using namespace std; int _tmain(int argc, _TCHAR* argv[]) { vector<string> myVector; myVector.push_back("Japan"); myVector.push_back("America"); myVector.push_back("England"); for (std::vector<string>::iterator it = myVector.begin() ; it != myVector.end(); ++it) { if(*it == "America") { //myVector.erase(it); // これだとnot incrementableエラーになる it = myVector.erase(it); // これが正しいコード } } for (std::vector<string>::iterator it = myVector.begin() ; it != myVector.end(); ++it) { cout << *it << endl; } } |
実行結果は以下の通りです。
1 2 |
Japan England |
注意点は、std::vector::eraseを実行すると、次のiteratorのポインターを返却するということです。
上記のコードの場合
it = myVector.erase(it);
ではなくて単に
myVector.erase(it);
として実行すると、以下のエラーが発生します。
Debug Assertion Failed!
Program: C:\Windows\system32\MSVCP110D.dll
File: c:\program files (x86)\microsoft visual studio
11.0\vc\include\vector
Line: 101Expression: vector iterator not incrementable
For information on how your program can cause an assertion
failure, see the Visual C++ documentation on asserts.
別のエラーサンプル
ちなみにvectorのメンバーを削除する場合、
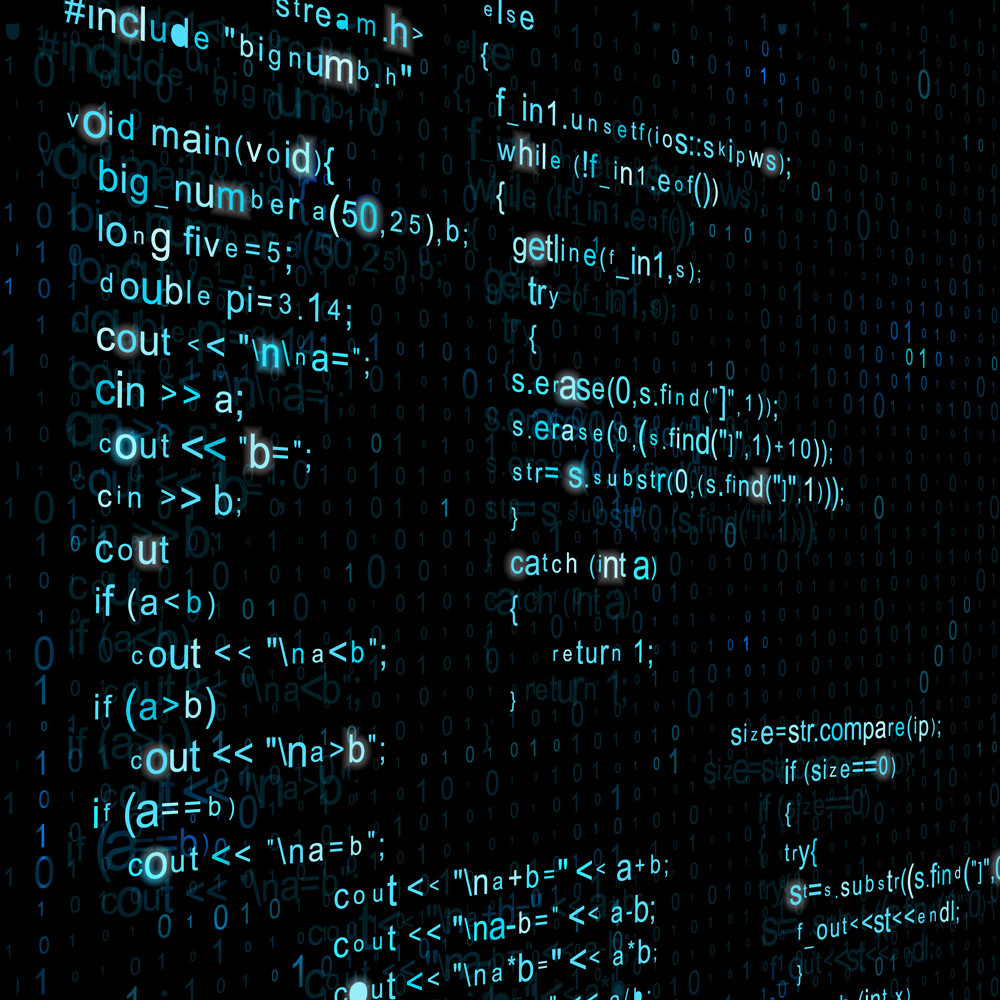
を真似た以下のサンプルでも動作しません。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include "stdafx.h" #include <stdint.h> #include <vector> #include <string> #include <iostream> using namespace std; int _tmain(int argc, _TCHAR* argv[]) { vector<string> myVector; myVector.push_back("Japan"); myVector.push_back("America"); myVector.push_back("England"); std::vector<string>::iterator it = myVector.begin(); while(it != myVector.end()) { if(*it == "America") { myVector.erase(it++); } else { ++it; } } for (std::vector<string>::iterator it = myVector.begin() ; it != myVector.end(); ++it) { cout << *it << endl; } } |
上記のプログラムを実行すると以下のエラーが表示されます。
Debug Assertion Failed!
Program: C:\Windows\system32\MSVCP110D.dll
File: c:\program files (x86)\microsoft visual studio
11.0\vc\include\vector
Line: 240Expression: vector iterator incompatible
For information on how your program can cause an assertion
failure, see the Visual C++ documentation on asserts.
上記のコードの場合でも
myVector.erase(it++);
ではなくて
it = myVector.erase(it);
とすれば正しく実行されます。
エラー(vector iterator not incrementable/incompatible)がアサーション(assertion)で表示されている
上記の二つのエラーサンプルがともにアサーション(assertion)で表示されている点に注意しよう。
Expression: vector iterator not incrementable
Expression: vector iterator incompatible
アサーションとは、プログラムの前提として満たされるべき条件をあらかじめ記述して、その条件を満たさない時に例外を発生させる機能です。
もしアサーション(assertion)を行っていないと、このエラーメッセージのようなわかりやすいダイアログを表示することがなく、プログラムがメモリ不正で異常終了することになります。
この場合は、コアダンプのようなファイルを解析して異常終了した原因を追跡することになります。
プロジェクトで自分で作るプログラムも極力アサーションを用意しておけば、不具合の起こりにくいプログラム、または、不具合原因を特定しやすいプログラムを作ることが可能になります。
その他エラーのメモ
ここで紹介した以外によく発生するエラーをまとめてみました。
エラーメッセージとして「Error: 識別子”cout”が定義されていません」と表示されることがあります。
対策として以下を入れてみてください。
#include <iostream>
using namespace std;
エラーメッセージとして「Error: 識別子”vector”が定義されていません」と表示されることがあります。
対策として以下を入れてみてください。
#include <vector>
using namespace std;
エラーメッセージとして「Error: これらのオペランドと一致する演算子”<<”はありません オペランドの型: std::ostream<<std::string」と表示されることがあります。
対策として以下を入れてみてください。
#include <string>
プログラミングの無料レッスン体験
約8,000名の受講生と80社以上の導入実績のあるプログラミングやWebデザインのオンラインマンツーマンレッスンCodecamp
<Codecampの特徴>
1 現役エンジニアによる指導
2オンラインでのマンツーマン形式の講義
3大手企業にも導入されている実践的なカリキュラム
↓無料体験レッスン実施中です。
コメント